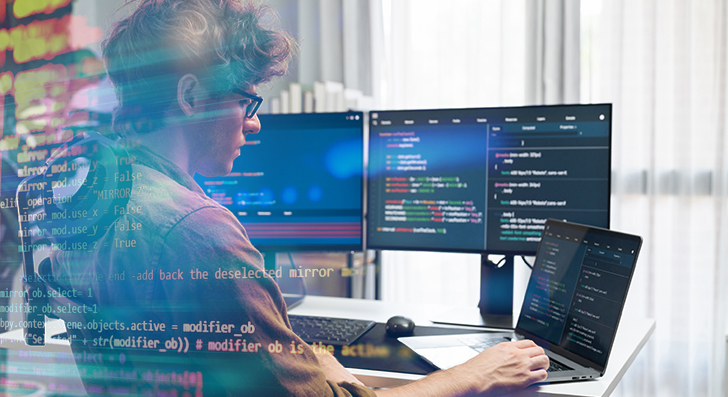
Scalability usually means your application can handle growth—additional end users, much more data, and more targeted visitors—with out breaking. To be a developer, making with scalability in mind will save time and anxiety afterwards. Below’s a clear and realistic guidebook that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability just isn't some thing you bolt on afterwards—it should be aspect of one's approach from the beginning. Lots of programs are unsuccessful once they improve quickly for the reason that the original structure can’t deal with the additional load. As a developer, you must think early about how your process will behave under pressure.
Start off by building your architecture to get adaptable. Steer clear of monolithic codebases wherever everything is tightly linked. As a substitute, use modular style or microservices. These designs split your application into smaller, impartial areas. Each individual module or services can scale on its own devoid of influencing the whole program.
Also, contemplate your databases from working day 1. Will it want to manage one million buyers or just a hundred? Choose the proper variety—relational or NoSQL—dependant on how your data will develop. Program for sharding, indexing, and backups early, Even though you don’t want them nevertheless.
A different significant position is to prevent hardcoding assumptions. Don’t write code that only will work beneath existing disorders. Take into consideration what would materialize Should your person foundation doubled tomorrow. Would your app crash? Would the databases decelerate?
Use design and style designs that support scaling, like message queues or celebration-driven programs. These assistance your application handle more requests without getting overloaded.
When you build with scalability in your mind, you are not just planning for success—you're reducing future problems. A very well-prepared program is easier to take care of, adapt, and improve. It’s superior to organize early than to rebuild later.
Use the Right Databases
Picking out the correct databases is often a essential Portion of developing scalable purposes. Not all databases are created the identical, and using the Completely wrong one can slow you down or maybe result in failures as your application grows.
Start out by knowing your data. Can it be hugely structured, like rows inside a desk? If Indeed, a relational databases like PostgreSQL or MySQL is an efficient match. These are definitely sturdy with relationships, transactions, and regularity. They also assist scaling methods like browse replicas, indexing, and partitioning to deal with extra targeted traffic and data.
When your details is much more adaptable—like user action logs, product catalogs, or paperwork—consider a NoSQL selection like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at dealing with substantial volumes of unstructured or semi-structured information and might scale horizontally more very easily.
Also, take into consideration your go through and produce patterns. Have you been accomplishing plenty of reads with less writes? Use caching and skim replicas. Are you handling a weighty write load? Explore databases which will handle large publish throughput, or simply event-primarily based facts storage units like Apache Kafka (for temporary information streams).
It’s also sensible to Assume in advance. You might not need Superior scaling characteristics now, but picking a databases that supports them suggests you received’t need to switch later.
Use indexing to speed up queries. Steer clear of needless joins. Normalize or denormalize your info dependant upon your entry designs. And constantly watch databases effectiveness when you improve.
Briefly, the appropriate databases will depend on your application’s framework, pace wants, And the way you anticipate it to develop. Consider time to pick wisely—it’ll help you save loads of issues later on.
Enhance Code and Queries
Quickly code is key to scalability. As your application grows, each individual smaller hold off adds up. Poorly penned code or unoptimized queries can decelerate functionality and overload your program. That’s why it’s vital that you Develop efficient logic from the beginning.
Start off by creating clean, very simple code. Prevent repeating logic and remove anything avoidable. Don’t select the most complicated solution if a straightforward a single works. Keep the features short, centered, and easy to check. Use profiling equipment to find bottlenecks—destinations in which your code takes far too extended to run or uses an excessive amount memory.
Up coming, look at your databases queries. These often sluggish things down in excess of the code itself. Ensure that Every question only asks for the data you really need. Keep away from SELECT *, which fetches almost everything, and rather decide on specific fields. Use indexes to speed up lookups. And stay clear of carrying out a lot of joins, especially across substantial tables.
In the event you observe the same info staying requested repeatedly, use caching. Retail outlet the results temporarily employing applications like Redis or Memcached so that you don’t really need to repeat high priced functions.
Also, batch your database operations if you can. In lieu of updating a row one after the other, update them in groups. This cuts down on overhead and helps make your app additional economical.
Make sure to test with huge datasets. Code and queries that operate high-quality with 100 records may crash whenever they have to manage one million.
Briefly, scalable applications are speedy applications. Keep the code limited, your queries lean, and use caching when needed. These actions assist your application remain easy and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to handle much more consumers and even more targeted traffic. If almost everything goes by way of one particular server, it is going to speedily become a bottleneck. That’s in which load balancing and caching are available in. These two tools help keep the application rapidly, steady, and scalable.
Load balancing spreads incoming site visitors across multiple servers. Instead of a person server accomplishing the many operate, the load balancer routes consumers to distinct servers according to availability. This means no one server will get overloaded. If 1 server goes down, the load balancer can send visitors to the Other individuals. Resources like Nginx, HAProxy, or cloud-based methods from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing information quickly so it could be reused rapidly. When users ask for the identical information yet again—like a product page or maybe a profile—you don’t must fetch it from the databases each time. You could serve it from the cache.
There are 2 typical sorts of caching:
1. Server-facet caching (like Redis or Memcached) retailers details in memory for rapidly access.
2. Consumer-facet caching (like browser caching or CDN caching) shops static documents close to the consumer.
Caching reduces database load, increases speed, and would make your app far more efficient.
Use caching for things that don’t improve usually. And normally ensure your cache is current when information does adjust.
In short, load balancing and caching are basic but impressive resources. Jointly, they assist your app take care of extra customers, keep speedy, and recover from troubles. If you propose to grow, you may need both of those.
Use Cloud and Container Resources
To create scalable apps, you need resources that allow your application improve easily. That’s exactly where cloud platforms and containers are available in. They provide you overall flexibility, cut down set up time, and make scaling much smoother.
Cloud platforms like Amazon Net Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and products and services as you would like them. You don’t have to purchase hardware or guess potential capability. When targeted traffic will increase, it is possible to insert additional means with just some clicks or quickly applying vehicle-scaling. When targeted visitors drops, you could scale down to economize.
These platforms also present expert services like managed databases, storage, load balancing, and stability instruments. You may center on constructing your app as opposed to handling infrastructure.
Containers are An additional key Software. A container offers your app and every thing it must operate—code, libraries, options—into a single unit. This can make it uncomplicated to move your app concerning environments, from the laptop to the cloud, without the need of surprises. Docker is the most well-liked Instrument for this.
When your application makes use of multiple containers, tools like Kubernetes assist you deal with them. Kubernetes handles deployment, scaling, and recovery. If a person portion of one's application crashes, it restarts it instantly.
Containers also make it straightforward to independent aspects of your app into services. You may update or scale elements independently, which is perfect for overall performance and trustworthiness.
In brief, applying cloud and container resources usually means it is possible to scale fast, deploy simply, and recover speedily when problems come about. If you want your app to mature without having restrictions, begin employing these tools early. They preserve time, cut down danger, and make it easier to stay focused on making, not correcting.
Keep track of Anything
If you don’t check your software, you received’t know when issues go Improper. Checking allows you see how your app is doing, location challenges early, and make much better choices as your application grows. It’s a critical part of developing scalable programs.
Start out by monitoring basic metrics like CPU usage, memory, disk Area, and response time. These let you know how your servers read more and companies are accomplishing. Applications like Prometheus, Grafana, Datadog, or New Relic can help you gather and visualize this info.
Don’t just keep an eye on your servers—keep an eye on your application far too. Regulate how much time it's going to take for customers to load webpages, how often mistakes take place, and the place they arise. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring inside your code.
Create alerts for crucial difficulties. By way of example, When your response time goes over a limit or a service goes down, you should get notified straight away. This allows you deal with difficulties rapidly, usually just before customers even notice.
Checking can be beneficial whenever you make changes. For those who deploy a different attribute and see a spike in faults or slowdowns, you may roll it back again prior to it results in authentic injury.
As your app grows, website traffic and info improve. Without the need of monitoring, you’ll miss indications of problems until it’s far too late. But with the correct applications in position, you stay on top of things.
In short, checking assists you keep the app trusted and scalable. It’s not nearly recognizing failures—it’s about knowing your procedure and ensuring it really works effectively, even stressed.
Last Feelings
Scalability isn’t just for massive companies. Even modest applications want a solid foundation. By coming up with cautiously, optimizing correctly, and utilizing the proper instruments, you are able to Make applications that expand efficiently without breaking under pressure. Get started little, Consider significant, and Construct clever.